- Published on
Tkinter Checkbox Tutorial - GUI with Python
- Authors
- Name
- jvip
- @PythonRoadmap
Table of Contents
Introduction to Checkbox Widget
A checkbox is a widget that looks like a square box, which toggles between two states, checked
and unchecked
. In Tkinter, a checkbox is created using the Checkbutton
widget. As mentioned, this widget allows us to make binary choices - either a checked or an unchecked state.
You must've already seen checkboxes in your everyday life. For example, you use a checkbox to accept Terms and Conditions on web pages.
Tkinter Checkbox Example
To create a checkbox in Tkinter, you need to import the Tkinter module and use the Checkbutton
class. Let's create a simple Tkinter application with a checkbox.
Create a new python file main.py
and write the following code :
import tkinter as tk
# Initialize the main window
root = tk.Tk()
root.title("Tkinter Checkbox Example")
# Variable to store the state of the checkbox
chk_state = tk.BooleanVar()
# Create a checkbox
chk = tk.Checkbutton(root, text="I accept Terms & Conditions", var=chk_state)
chk.pack()
# Start the GUI event loop
root.mainloop()
This is a basic example of how to create a Tkinter Checkbox using the Checkbutton
class. Let's see the output :
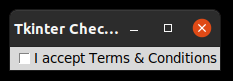
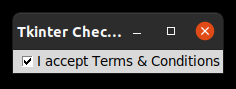
In the code, we created a BooleanVar
object to store the state of the checkbox. Everytime the state of our checkbox is changed, the value of the BooleanVar
will be changed as well.
Tkinter Checkbox Configuration
For this tutorial, we only configured the checkbox text, and output variable to keep the example simple. You can configure many more options in a Tkinter Checkbox, as follows :
text
: Set the label of the checkbox. In our example, we set the text toI accept Terms & Conditions
.variable
: Set a Tkinter variable to store the checkbox's state. In our example, we set the variable tochk_state
.onvalue
andoffvalue
: Define custom values that the linked variable will store when the checkbox is checked or unchecked.command
: A callback python function, this will be called every time the checkbox's state changes.
Tkinter Checkbox Command/Callback
Let's see an example of the callback configuration in the Tkinter Checkbox.
import tkinter as tk
# Initialize the main window
root = tk.Tk()
root.title("Tkinter Checkbox Example")
# Variable to store the state of the checkbox
chk_state = tk.BooleanVar()
def show_state():
print(chk_state.get())
# Create a checkbox
chk = tk.Checkbutton(root, text="I accept Terms & Conditions", var=chk_state)
chk.config(command=show_state)
chk.pack()
# Start the GUI event loop
root.mainloop()
In this example, every time the checkbox if checked or unchecked, the callback function show_state
will be called. This function will print whatever value is stored in chk_state
.
Conclusion
This post is part of a series of posts that will explain how to use Tkinter in Python GUI Programming. In this blog post we learnt how to use Tkinter's Checkbox Widget. We also learned about the different configuration options, and we saw how to use the callback function to print the state of the checkbox.
If you love this small tutorial, please give us a share or follow on Twitter.
Feel free to comment if you have any questions or suggestions. As always, keep learning 🤞