Flatten List of Lists (Nested List) in Python
Learn how can you flatten a nested list to a flat list of individual elements in Python. We will be sharing 5 ways to do this.
What do we mean by "Flattening"
Often times we may get to work on nested list or list of lists type of data, and we need to flatten it. Flattening means converting a list of list of elements to list of elements.Or in other words, converting a multi-dimensional list to a one-dimensional list.
Let's understand this by an example :
multi_dimensional_list = [
[1, 2, 3, 4],
["a", "b", "c"],
[5, 6, 7, 8],
]
This was an example of a multi dimensional list, or a list of lists. Now let's see an example for flattened version of the same list :
flattened_list = [1, 2, 3, 4, "a", "b", "c", 5, 6, 7, 8]
Different ways to flatten a nested list in Python
There are various ways to flatten a nested list, using built-in features and with third party libraries. Some of them are :
- Flattening with a For loop
- Flattening using List Comprehension
- Flattening with Recursion
- Flattening with
sum()
- Flattening with
itertools
- Flattening with Third party libraries such as
more_itertools
andfuncy
Let's take a look at each methods, one by one.
How to flatten list of lists with for loop
In this example, we will :
- Iterate on the list
nested_list
- And we will extend the
flattened_list
with the sublist - After a complete iteration on
nested_list
, we will have a flattened list
Copy the below code, and save it in a file main.py
:
nested_list = [[1, 2, 3], [4, 5], [6, 7, 8]]
flattened_list = []
for sublist in nested_list:
flattened_list.extend(sublist)
print(flattened_list)
Output :
[1, 2, 3, 4, 5, 6, 7, 8]
Note : While this approach is simple and works well for 1-level nested list. It can be inefficient for large lists and incorrect for deeply nested lists.
For cases like this, it makes more sense to use recursion or libraries like
more-itertools
. You can find guides for both below.
Flattening list of lists with List Comprehension
Another short-hand to our for loop approach is using Python list comprehensions for this task. Let's see with an example :
nested_list = [[1, 2, 3], [4, 5], [6, 7, 8]]
flattened_list = [item for sublist in nested_list for item in sublist]
print(flattened_list)
Output :
[1, 2, 3, 4, 5, 6, 7, 8]
While this does the job in a one-liner, and looks cleaner - it's not the most optimal way to flatten as we require two iterations here.
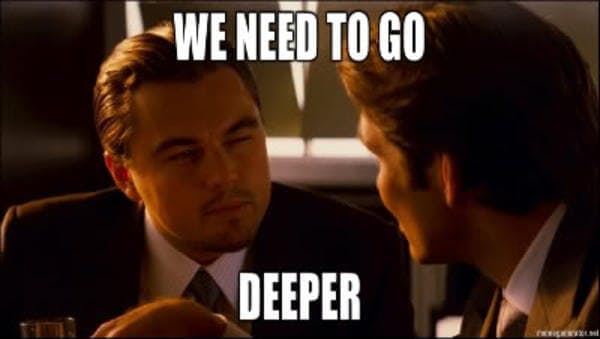
Flattening nested lists with Recursion
With recursion, we can flatten deeply nested lists as well. Let's see with an example, consider we have a deeply nested list like this :
nested_list = [1, [2, [3, 4], 5], 6]
For cases like this, we need to keep flattening it till we no longer encounter list of elements again. Let's write code for flattening list with recursion :
def flatten(input_list):
result = []
for item in input_list:
if isinstance(item, list):
result.extend(flatten(item))
else:
result.append(item)
return result
nested_list = [1, [2, [3, 4], 5], 6]
flattened_list = flatten(nested_list)
print(flattened_list)
Output :
[1, 2, 3, 4, 5, 6]
The recursive approach is more clean and elegant as compared to the previous approach. And is capable of flattening deeply-nested lists as well.
Flattening list of lists with sum()
method
You can use python's built-in sum()
method to flatten a list of lists as well. When we use sum()
method on a list of lists, it concatenates all the sub-lists together to form a list of elements.
nested_list = [[1, 2, 3], [4, 5], [6, 7, 8]]
flattened_list = sum(nested_list, [])
print(flattened_list)
Output :
[1, 2, 3, 4, 5, 6, 7, 8]
While this is more of a hacky way to do it, it still does the job.
Flattening nested list with itertools
library
itertools
is part of Python Standard library, which has methods to flatten lists as well. We can flatten list of lists in Python using itertools.chain
function.
The chain
method takes a list of Python iterables and returns a single grouped iterable. This behaviour of chain
method helps us to flatten our list.
Let's see an example :
from itertools import chain
nested_list = [[1, 2, 3], [4, 5], [6, 7, 8]]
flattened_list = list(chain.from_iterable(nested_list))
print(flattened_list)
Output :
[1, 2, 3, 4, 5, 6, 7, 8]
Using
itertools.chain
method is recommended for working with large or deeply nested lists. It's efficient and very clean way to flatten a nested list.
Flattening with Third party libraries
You can also flatten nested lists using third party libraries in Python. We personally recommend using itertools.chain
, as it's clean enough already. We will be sharing examples of two libraries :
more-itertools
funcy
Flatten nested lists with more-itertools
more-itertools
library as the name suggests, provides more methods that are missing from itertools
. It has methods to flatten nested lists as well.
-
Install
more-itertools
library using pip :pip install more-itertools
-
Example code :
from more_itertools import flatten nested_list = [[1, 2, 3], [4, 5], [6, 7, 8]] flattened_list = list(flatten(nested_list)) print(flattened_list)
Flatten nested lists with funcy
funcy
library provides various functional programming methods, including methods to flatten nested lists.
-
Install
funcy
library using pip :pip install funcy
-
Example code :
from funcy import flatten nested_list = [[1, 2, 3], [4, 5], [6, 7, 8]] flattened_list = list(flatten(nested_list)) print(flattened_list)
Conclusion
And that's all! In this post, we saw various ways and examples to flatten nested lists in Python. Remember to pick the method that's more aligned with your project's coding style and if efficient enough for your use case.
If you love this small tutorial, please give us a share or follow on Twitter.
Get in Touch